Spring Boot - Swagger 2 Set up
Lets learn how to set up Swagger for a Spring Boot application.
Swagger makes it easy to document Restful API. You can visit Swagger’s Homepage to get more information about Swagger.
Maven
First need to add maven dependency for springfox-swagger2. Springfox is an implementation for Swagger.
1 | <dependency> |
Java Configuration
You can enable Swagger using @EnableSwagger2 annotation
1 |
|
Here Docket is a builder which is intended to be the primary interface into the Springfox framework. Provides sensible defaults and convenience methods for configuration.
Here are the methods used
- Docket.select() - returns an instance of ApiSelectorBuilder to give fine grained control over the endpoints exposed via swagger.
- ApiSelectorBuilder.apis() - allows selection of RequestHandler’s using a predicate. The example here uses an any predicate (default). Out of the box predicates provided are any, none, withClassAnnotation, withMethodAnnotation and basePackage.
- ApiSelectorBuilder.paths() - allows selection of Path’s using a predicate. The example here uses an any predicate (default). Out of the box Springfox provide predicates regex, ant, any, none.
- ApiSelectorBuilder.build() - builds a Docket
RequestHandlerSelectors and PathSelectors are both predicates. You can use any() for both. You can customize by selecting the package used or path.
Swagger Usage
All Swagger annotations are documented here.
Controller with Swagger API
1 |
|
Swagger UI Url will be http://localhost:8080/context-root/swagger-ui.html. In our case, the Url will be http://localhost:8080/swagger-ui.html
API Info
You can set the API Info for the API. These information will appear in the UI.
1 |
|
Response Customization
You can customize the Response Message section. Here is the example
1 |
|
The Resulting API:
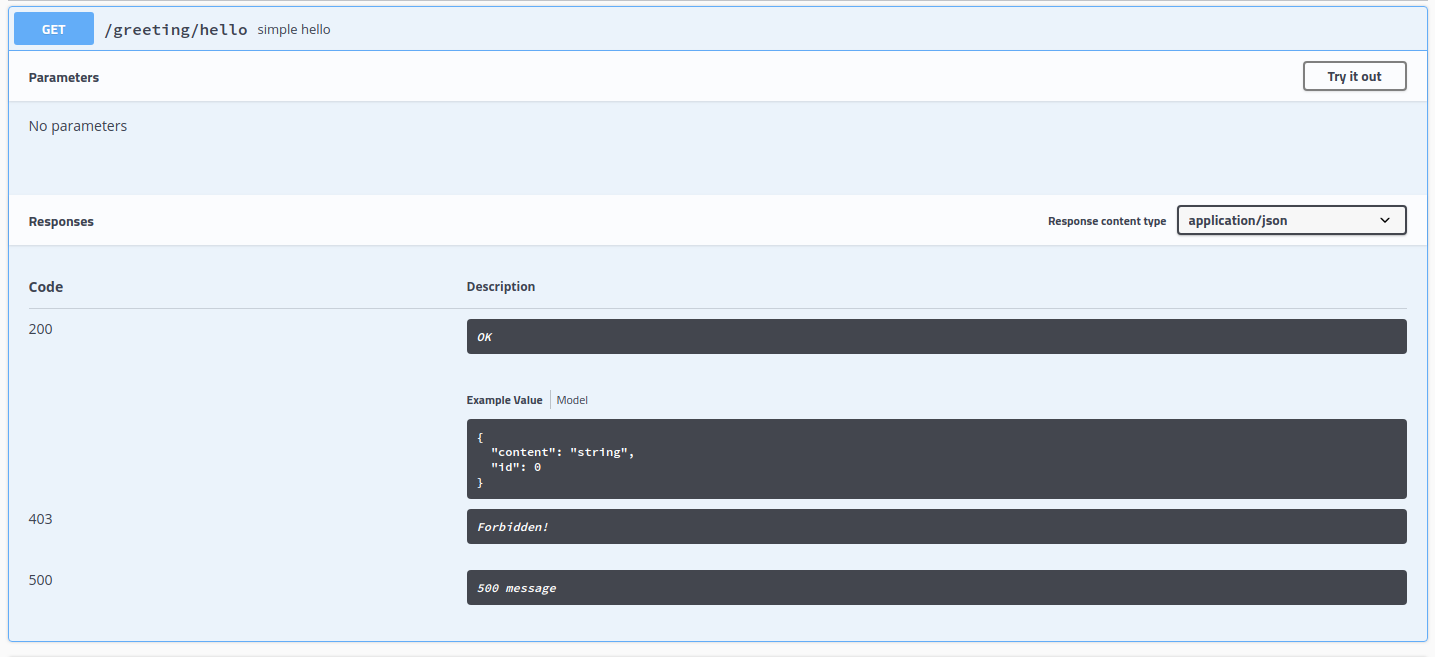
Reference
- baeldung - Setting Up Swagger 2 with a Spring REST API
- Spring Boot 2 RESTful API Documentation With Swagger 2 Tutorial
For more information on Springfox, see
Sourcecode - SpringBootExamples - web-application