Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications.
It uses YAML files to configure application’s services.
Service
Services
are really just “containers in production.”. A Service runs one image and it defines ports, volumes, resource limitation, restart policy and replicas.
Installation
Instruction to install Compose is here
To install in Linux
1 | # download latest version of Docker Compose |
Simple Example
Here is the example from Get Started with Docker Compose
Files Structure
1 | composetest/ |
app.py
1 | import time |
requirements.txt
1 | flask |
Dockerfile
1 | FROM python:3.4-alpine |
docker-compose.yml - defines two service, web and redis.
1 | version: '3' |
use docker-compose up -d
to start the services
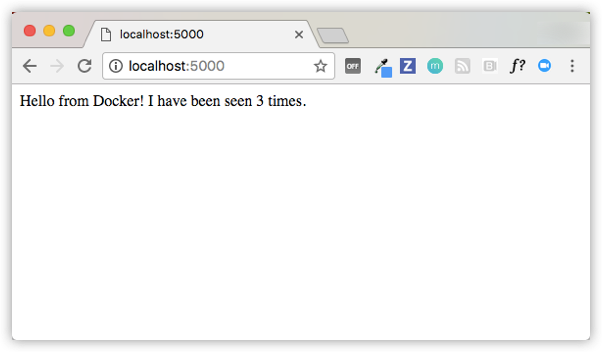
Common docker-compose Commands
docker-compose up -d
is the most used command to start service in the background
1 | $ docker-compose up -d |
The default compose file to be used is docker-compose.yml, you can set alternative file using -f
option.
1 | docker-compose -f docker-compose.dev.yml up -d |
use docker-compose ps
to list containers. If a container is not UP, you can usually use docker log
command to see if there is error starting the container.
1 | $ docker-compose ps |
Use docker-compose build
command to rebuild the containers. This is useful after a change to a service
1 | $ docker-compose build |
use docker-compose stop
to stop the containers without removing them. You can use docker-compose start
to start the services again
1 | $ docker-compose stop |
use docker-compose down
to stop containers and removes containers, networks created by up. use -v
to remove volumes and use --rmi
to remove image
1 | $ docker-compose down -v --rmi all |
Print out log messages from all container.
1 | docker-compose logs -f --tail=0 |
Use docker help
command to display the help menu.
For all the command reference, see docker-compose command-line Reference
docker-compose.yml file
Compose file has a version number. current version is version 3.
You can include the version number at the top of docker-compose.yml
1 | version: '3' |
Service Configuration
Then services
list all the services to create.
build - usually a path to a build context. It can also specify context and optionally Dockerfile and args.
1 | version: '3' |
image - Specify the image to start the container from.
1 | image: "redis:alpine" |
environment - sets environment variable
1 | environment: |
ports - specify ports(Host:Container)
1 | ports: |
1 | volumes: |
depends_on - sets dependency between services.
1 | services: |
Restart - sets restart policy. Default is “no”
1 | restart: "no" |
Volume Configuration
you can create named volumes in compose file
Example
1 | version: "3" |
Network Configuration
by default, Compose set up a bridge network for your app. You can customize it.
1 | networks: |
Other Examples
Running Postgres and Adminer
Two services will be started. postgres database and adminer
1 | # Use postgres/example user/password credentials |
example from https://hub.docker.com/_/postgres
Wordpress
1 | version: '3' |